How to Create a Discord Bot to Read Channel Content and Post to Another Channel
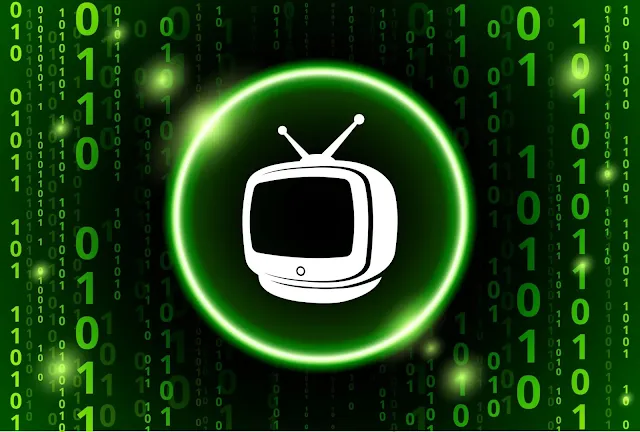
Introduction:
Discord is a popular platform for communication among gamers, communities, and various interest groups. Discord bots can enhance the functionality of your server by automating tasks and providing additional features. In this article, we will guide you through the process of creating a Discord bot that reads messages from specific channels and posts them to another channel, allowing you to log or share important messages seamlessly.
1. Prerequisites:
Before we dive into creating the Discord bot, ensure you have the following:Python installed on your computer.
- A Discord account.
- A Discord server where you have administrative privileges.
- A text editor or IDE for writing and editing code.
2. Creating a Webhook:
- Inside your Discord server, right-click the channel where you want to post messages and select "Edit Channel."
- In the channel settings, go to the "Webhooks" tab and click "Create Webhook." Provide a name and, optionally, an avatar for your webhook.
- Click "Copy Webhook URL" to save the webhook URL to your clipboard. This URL allows your bot to send messages to the channel.
3. Implementation:
import websocket
import json
import threading
import time
import os
import sys
import requests as rq
These lines import necessary Python libraries and modules. websocket and requests are used for handling WebSocket connections and making HTTP requests, respectively.
module_path = os.path.abspath(os.getcwd())
if module_path not in sys.path:
sys.path.append(module_path)
Here, the script ensures that the current working directory (os.getcwd()) is added to the Python path to allow importing modules from this directory.
# Configurations Sections
discord_token = "MTAxNTM1MDL3xGlf51m9s"
source_channel_ids = '114876314,114721474'
webhook_target = "https://discorbuJcwtk1ylI7x9nv1F5t6"
This section contains configuration variables:discord_token: Your Discord bot's token, which you should replace with your actual bot token.
source_channel_ids: A string containing the IDs of the channels you want to monitor. These IDs should be separated by commas.
webhook_target: The URL of the webhook where the bot will post messages.
def send_json_request(ws, request):
ws.send(json.dumps(request))
This function, send_json_request, takes a WebSocket connection (ws) and a JSON request object (request) as parameters. It sends the JSON request to the WebSocket connection after converting it to a string.
def receive_json_response(ws):
response = ws.recv()
if response:
return json.loads(response)
The receive_json_response function takes a WebSocket connection (ws) as a parameter. It receives a JSON response from the WebSocket, parses it, and returns the parsed JSON object.
def heartbeat(interval, ws):
print('Heartbeat Activated')
while True:
time.sleep(interval)
heartbeatJSON = {
"op": 1,
"d": "null"
}
send_json_request(ws, heartbeatJSON)
print("Heartbeat Activated - Looking for information.")
This function, heartbeat, sends periodic heartbeat messages to the Discord gateway to maintain the connection. It runs in a loop with a specified interval. The function sends a JSON object with operation code 1 and a null payload to the WebSocket using send_json_request.
ws = websocket.WebSocket()
ws.connect("wss://gateway.discord.gg/?v=6&encording=json")
Here, a WebSocket connection ws is created and connected to the Discord gateway using the specified URL.
event = receive_json_response(ws)
heartbeat_interval = event['d']['heartbeat_interval'] / 1000
threading._start_new_thread(heartbeat, (heartbeat_interval, ws))
This section receives an initial event from the Discord gateway and extracts the heartbeat_interval from it. It then starts a separate thread running the heartbeat function to maintain the WebSocket connection.
payload = {
"op": 2,
"d": {
"token": discord_token,
"properties": {
"$os": 'windows',
'$browser': 'chrome',
'$device': 'pc'
}
}
}
send_json_request(ws, payload)
This code sends an initial payload to the Discord gateway with the bot's token and other properties like the operating system, browser, and device information.
id_list = source_channel_ids.split(',')
while True:
event = receive_json_response(ws)
try:
channel_id = event['d']['channel_id']
if (str(channel_id) in id_list) and event['t'] == 'MESSAGE_CREATE':
result = rq.post(webhook_target, data=json.dumps(event['d']),
headers={"Content-Type": "application/json"})
status_code = result.status_code
if status_code == 204:
print("Message Inserted into Target channel successfully")
print("Request Status code is: " + str(status_code))
except:
pass
In this final section, the script enters an infinite loop to continuously listen for events from the Discord gateway. When a message creation event ('MESSAGE_CREATE') is detected in one of the specified channels, it posts the message to the target channel using the specified webhook URL. The script also prints the status code of the HTTP request made to the webhook.
This detailed explanation breaks down the provided code step by step, helping you understand how it functions to create a Discord bot that reads and forwards messages between channels.